# 12.10 拖拽列表
国内美居 可拖拽排序组件
APP内置组件,可直接在template中使用,不需要import导入和注册, 该组件从8.17版本开始生效。
使用规则
1、通过列表前的“+”“-”按钮,实现两个不同列表模块的增减。
2、通过拖拽手势,实现列表项增减:
拖拽开始时效果:点击内容项进入选中状态,区域背景发现颜色变化
过程中的效果:长按拖动内容项可支持垂直移动
结束的效果:手指移开内容项时,取消选中区域背景,背景颜色恢复初始颜色
2、通过拖拽手势,实现列表项增减:
拖拽开始时效果:点击内容项进入选中状态,区域背景发现颜色变化
过程中的效果:长按拖动内容项可支持垂直移动
结束的效果:手指移开内容项时,取消选中区域背景,背景颜色恢复初始颜色
# 实例 :
拖拽列表
扫码预览
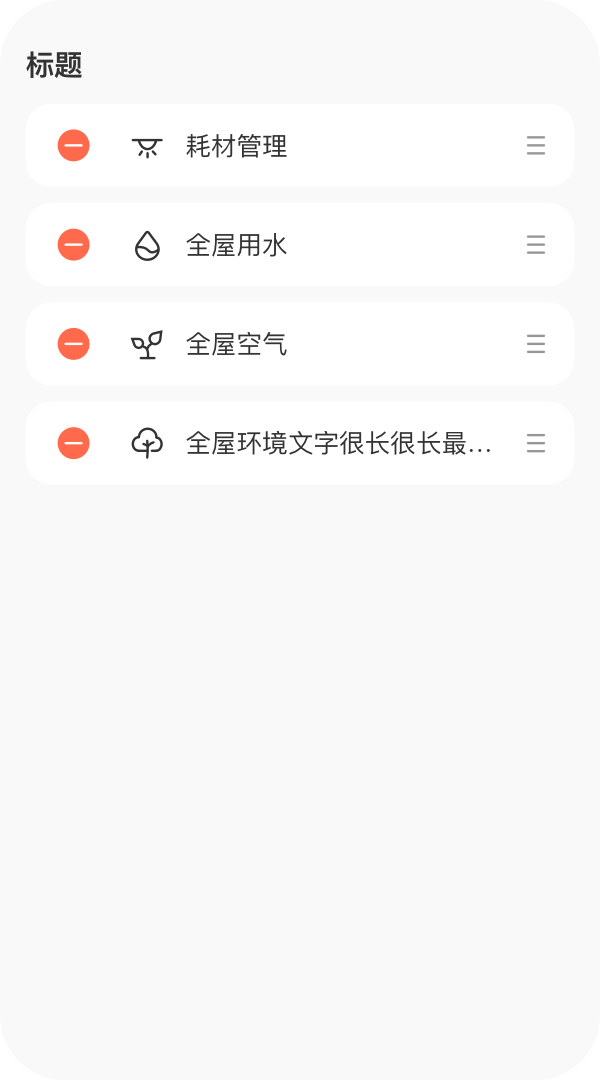
# 基础用法
<template>
<div class="wrapper">
<dof-minibar
:title="title"
backgroundColor="transparent"
textColor="#000000"
:middle-text-style="headerStyle"
@dofMinibarLeftButtonClicked="minibarLeftButtonClick"
@dofMinibarRightButtonClicked="minibarRightButtonClick"
>
<div slot="left">
<image :src="leftButton" style="height: 55px;width: 55px;transform:translateX(-10px);"></image>
</div>
<div slot="right" class="right-img-wrapper">
<image :src="rightButton" style="height: 32px;width: 32px;"></image>
</div>
</dof-minibar>
<dof-button
class="center margin-top-80"
text="切换编辑状态"
type="primary"
size="big"
@dofButtonClicked="siwtchEdit"
></dof-button>
<drag-vertical-list-view
class="seekbar"
:data="listData"
@onDeleteEvent="onDeleteEvent"
@onDragEndEvent="onDragEndEvent"
@onClickEvent="onClickEvent"
>
</drag-vertical-list-view>
</div>
</template>
<script>
import { DofMinibar, DofButton } from 'dolphin-weex-ui'
/*
onDeleteEvent 删除给删除的id, 排序后点击完成给list所有的数据
onDragEndEvent 排序后给list数据
onClickEvent 给当前item数据
*/
export default {
components: {
DofMinibar,
DofButton
},
data() {
return {
leftButton: './assets/image/header/back_black@2x.png',
rightButton: './assets/image/header/refresh.png',
headerStyle: {
fontFamily: 'PingFangSC-Regular',
fontWeight: '900',
fontSize: '28px',
letterSpacing: 0
},
// editing: true,
// src: 'https://dsdcp.smartmidea.net/mcsp/prod/20230315/0f4fea7232b34be4a123b93e97a87278.pdf',
title: '拖拽tableview',
listData: {
backgroundColor: '#F9F9F9', //组件背景色 默认为 #F9F9F9
isEditing: false, //是否编辑
rowHeight: 60, //行高 默认为52,建议大于等于52,参考UI
rowSpace: 10, //行间距 默认为10
itemTextColor: '#333333', //默认字体颜色#333333
itemBgColor: '#FFFFFF', //行背景色 #FFFFFF
itemFont: 16, //字体大小 默认为16
itemCornerRadius: 16, //默认为16,参考UI(注意:android系统0-rowHeight/2才有效果)
iconCornerRadius: 0, //默认为0
iconHeight: 32, //图片高度,默认32, 图片宽度等于高度,参考UI
deleteIconHeight: 32, //图片高度,默认32, 图片宽度等于高度,参考UI
sortIconHeight: 32, //图片高度,默认32, 图片宽度等于高度,参考UI
deleteIcon: '', //默认用切图 删除图片
sortIcon: '', //默认用切图 排序图片
list: [
{
itemId: '0',
name: '全屋照明', //名称
icon: 'https://pic.5tu.cn/uploads/allimg/1708/pic_5tu_big_201708111325384841.jpg' //icon,必传
},
{
itemId: '1',
name: '全屋用水',
icon: 'https://img1.baidu.com/it/u=413643897,2296924942&fm=253&fmt=auto&app=138&f=JPEG?w=800&h=500'
},
{
itemId: '2',
name: '全屋空气',
icon: ''
},
{
itemId: '3',
name: '全屋环境文字很长很长最多一行点点...',
icon: ''
},
{
itemId: '4',
name: '洗衣机',
icon: ''
},
{
itemId: '5',
name: '郑二',
icon: ''
},
{
itemId: '6',
name: '张三',
icon: ''
},
{
itemId: '7',
name: '王四',
icon: ''
},
{
itemId: '8',
name: '蛋黄酱'
},
{
itemId: '9',
name: '番茄酱',
icon: ''
},
{
itemId: '10',
name: '大冬瓜',
icon: ''
},
{
itemId: '11',
name: '大狮子', //名称
icon: 'https://pic.5tu.cn/uploads/allimg/1708/pic_5tu_big_201708111325384841.jpg' //icon,必传
},
{
itemId: '12',
name: '大象',
icon: 'https://img1.baidu.com/it/u=413643897,2296924942&fm=253&fmt=auto&app=138&f=JPEG?w=800&h=500'
},
{
itemId: '13',
name: '水牛',
icon: ''
},
{
itemId: '14',
name: '香蕉', //名称
icon: 'https://pic.5tu.cn/uploads/allimg/1708/pic_5tu_big_201708111325384841.jpg' //icon,必传
},
{
itemId: '15',
name: '苹果',
icon: 'https://img1.baidu.com/it/u=413643897,2296924942&fm=253&fmt=auto&app=138&f=JPEG?w=800&h=500'
},
{
itemId: '16',
name: '香梨',
icon: ''
}
]
}
}
},
computed: {},
methods: {
onDeleteEvent(event) {
this.$alert(event)
},
onDragEndEvent(event) {
this.$alert(event)
},
onClickEvent(event) {
this.$alert(event)
},
minibarLeftButtonClick() {},
minibarRightButtonClick() {
this.$reload()
},
siwtchEdit() {
this.listData.isEditing = !this.listData.isEditing
this.$toast('啦啦啦')
this.listData = {
...this.listData
}
}
}
}
</script>
<style scoped>
.wrapper {
width: 750px;
background-color: aliceblue;
}
.margin-top-80 {
margin-top: 40px;
}
.seekbar {
/* width: 600px; */
margin-left: 40px;
margin-right: 40px;
height: 1000px;
/* right: 200px; */
margin-top: 20px;
}
</style>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
# Attributes: data
Prop | Type | Required | Default | Description |
---|---|---|---|---|
backgroundColor | String | N | #F9F9F9 | 组件背景色 默认为 #F9F9F9 |
isEditing | Boolean | N | false | 是否编辑 |
rowHeight | Number | N | 52 | 行高 默认为52,建议大于等于52,参考UI |
rowSpace | Number | N | 10 | 行间距 默认为10 |
itemTextColor | String | N | #333333 | 默认字体颜色#333333 |
itemBgColor | String | N | #FFFFFF | 行背景色 #FFFFFF |
itemFont | Number | N | 16 | 字体大小 默认为16 |
itemCornerRadius | Number | N | 25 | 默认为16,参考UI 行圆角 |
iconCornerRadius | Number | N | 5 | 默认为0 图片圆角 |
deleteIconHeight | Number | N | 32 | 删除图片高度,默认32,图片宽度等于高度,参考UI |
sortIconHeight | Number | N | 32 | 排序图片高度,默认32, 图片宽度等于高度,参考UI |
deleteIcon | String | N | - | 默认用切图 删除图片 |
sortIcon | String | N | - | 默认用切图 排序图片 |
list | Array | Y | [] | 列表数据 |
iconBgColor | String | Y | #0000ff | 图片背景色 8.23新增 |
# Events
事件名称 | 说明 | 回调参数 |
---|---|---|
onClickEvent | 点击事件通知 返回当前点击的item数据 | {"itemId": '', "name": '', "icon": ''} |
onDeleteEvent | 删除事件通知 返回当前点击的itemId | {"itemId": ''} |
onDragEndEvent | 拖拽结束 返回当前list数据 | list数据返回 |
← 12.9 拖拽